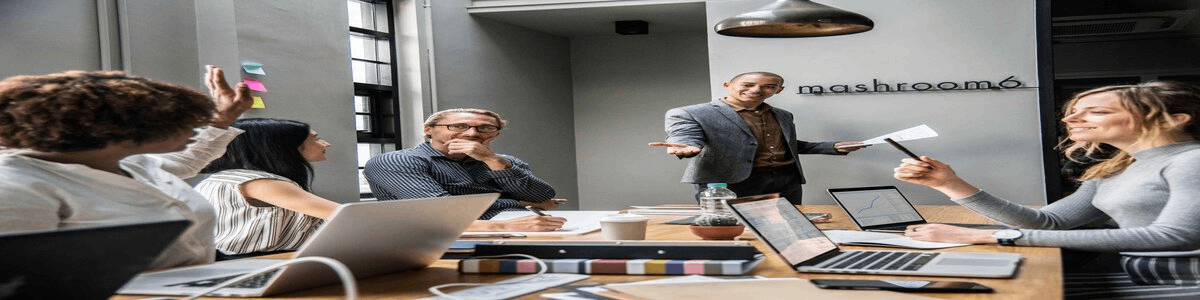
Category: JavaFX Script
-
Binding Java Objects in JavaFX Script
Bindings are one of the key features of the JavaFX language, because they simplify one´s life tremendously. By reducing the…
-
JavaFX is out – or thinking about my last year with JavaFX
It’s been done. Today the first version of the JavaFX SDK was released. What better occasion could there be to…
-
Creating JavaFX sequences in Java programs
The last article explained, how sequences are represented in the runtime and touched some of the possibilities to create new…
-
Internal design of JavaFX sequences
This is the first part in a series of articles about JavaFX sequences. It will focus on the basic concepts…
-
Refactoring a function to become a bound function
The specification for bound functions seems to be very strict at first sight. But in this article, I present a…
-
Creating JavaFX objects in Java programs
So far, all of the examples in my previous article about how to use JavaFX objects in Java code expected…
-
Using JavaFX sequences in a Java program
The last two articles were about how to pass and deal with primitive datatypes, Java objects, and JavaFX objects. This…
-
Using JavaFX objects in Java code
As shown in the last article, passing parameters between a JavaFX script and Java code is fairly simple, if the…
-
Writing Java code for JavaFX Script
When writing Java classes which can be used in JavaFX scripts, three factors have to be considered: how to create…